In this exercise you will model the collision between two cars that are constrained to move along a linear track. The blue car (on the left) has a spring attached to it’s right end that can collide with the red car.
The complicated task of determining how much the spring is compressed and drawing the spring at the proper location with the proper length is taken care of for you. You just need to include code to compute the spring force, update the momentum and position of each car, and compare the total momentum of the system before the collision to the total momentum after the collision. Try various scenarios where you change the masses of the gliders, the spring constant, or the initial conditions of each car. You will likely find some situations where the model will fail because the collision is too forceful and the spring becomes fully compressed. In reality, when this happens, the forces on the cars will be significantly larger than the spring force you will compute. This model as currently written doesn’t account for this type of collision so you will probably observe the cars passing though each other. Select a good set of parameters and initial conditions that clearly shows the total momentum of the system being conserved and submit the URL for this program for grading.
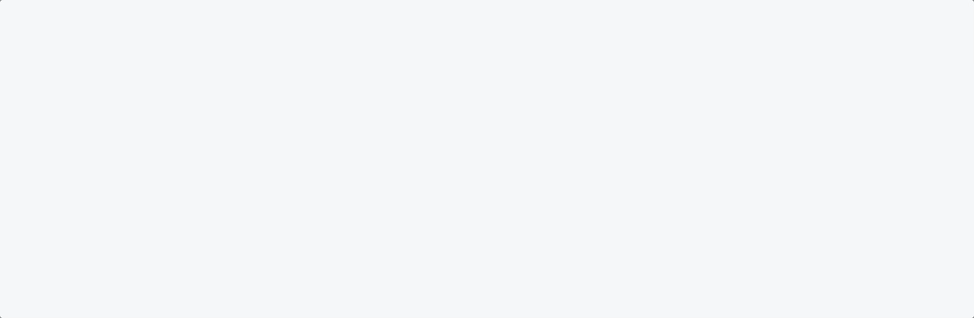
# # 03-C One Dimensional Collision # This program draws a horizontal track and two cars. The left (BLUE) car # has a spring attached to its right end and can collide with the RED car # on the right. # Set up the scene. First, modify the size and shape of the canvas to suit # a long track with two carts. scene.height = 400 scene.width = 1000 # Now, change the background color so the spring will be more easily visible. scene.background = vector(0.9, 0.9, 0.9) # Create a track that is 2 cm high, 10 cm across and 1.6 m long # Position the track just a little below the xz plane so that when the # cars move along the x-axis (y coordinate of zero) they will appear to be # on top of the track. track = box(pos=vector(0,-0.025,0), height=0.02, length=1.6, width=0.10, color = vector(0.5, 0.5, 0.5)) # For the following code (in the loop) to work properly, car1 must be given an # initial position that is to the LEFT of car2 and they must be given initial # velocities such that they will hit each other. # Create a BLUE car 3cm high, 12 cm long, and 6 cm wide. car1 = box(height=0.03, length=0.12, width=0.06, color=color.blue) # A - Set the mass, initial position, velocity, and momentum of the Blue car. car1.m = 0.5 car1.pos = vector(-0.25, 0, 0) car1.v = vector(0, 0, 0) car1.p = car1.m * car1.v # Create a RED car 3cm high, 12 cm long, and 6 cm wide. car2 = box(height=0.03, length=0.12, width=0.06, color=color.red) # B - Set the mass, initial position, velocity, and momentum of the Red car. car2.m = 1.0 car2.pos = vector(0.75, 0, 0) car2.v = vector(0, 0, 0) car2.p = car2.m * car2.v # set the spring constant (k) and unstretched length (L0) of the spring. k = 200 L0 = 0.04 #Create a helix to represent the spring. Draw it at the right end of the Blue car. spring = helix(coils=10, color=color.black) spring.radius = 0.01 spring.axis = vector(L0, 0, 0) spring.pos = car1.pos + car1.axis/2 # Setup the momentum graphs MyGraph = graph(title = 'Momentum', width = 1000, height = 400, xtitle = 'Time, t (s)', ytitle='Momentum, p (kg m/s)', fast = False) Car1_Momentum = gcurve(color = color.blue, width = 2, label = 'Car 1 (Left)') Car2_Momentum = gcurve(color = color.red, width = 2, label = 'Car 2 (Right)') Total_Momentum = gcurve(color = color.green, width = 2, label = 'Total Momentum') # Setup an elapsed time (t) and an increment (dt) in seconds t = 0 dt = 0.01 # C - Print the total momentum of the system consisting of the blue and red cars. # Solve iteratively as long as both cars are on the track. while((abs(car1.pos.x) < track.axis.x/2) and (abs(car2.pos.x) < track.axis.x/2)): # Slow the loop for animation rate(100) # If the carts are close enough to each other then shorten the spring springlength = min(L0,abs(car2.pos.x - car2.axis.x/2 - (car1.pos.x + car1.axis.x/2))) # Compute the vector L as defined in the text L = vector(springlength,0,0) # D - Compute the stretch (s) of the spring # E - Compute the force exerted on the Red car by the spring. # F - update the momentum and then the position of the Red car. # G - Compute the force exerted on the Blue car by the spring. # H - update the momentum and then the position of the Blue car. # Redraw the spring at its new location. spring.axis = L spring.pos = car1.pos + car1.axis/2 # Advance the elapsed time counter t = t + dt # Update the Plots Car1_Momentum.plot(t, car1.p.x) Car2_Momentum.plot(t, car2.p.x) Total_Momentum.plot(t, car1.p.x + car2.p.x) # I - Print the final value of the total momentum of the system.